CalendarioVer3.java
Java
Publicado el 23 de Diciembre del 2016 por Jose Miguel (9 códigos)
3.021 visualizaciones desde el 23 de Diciembre del 2016
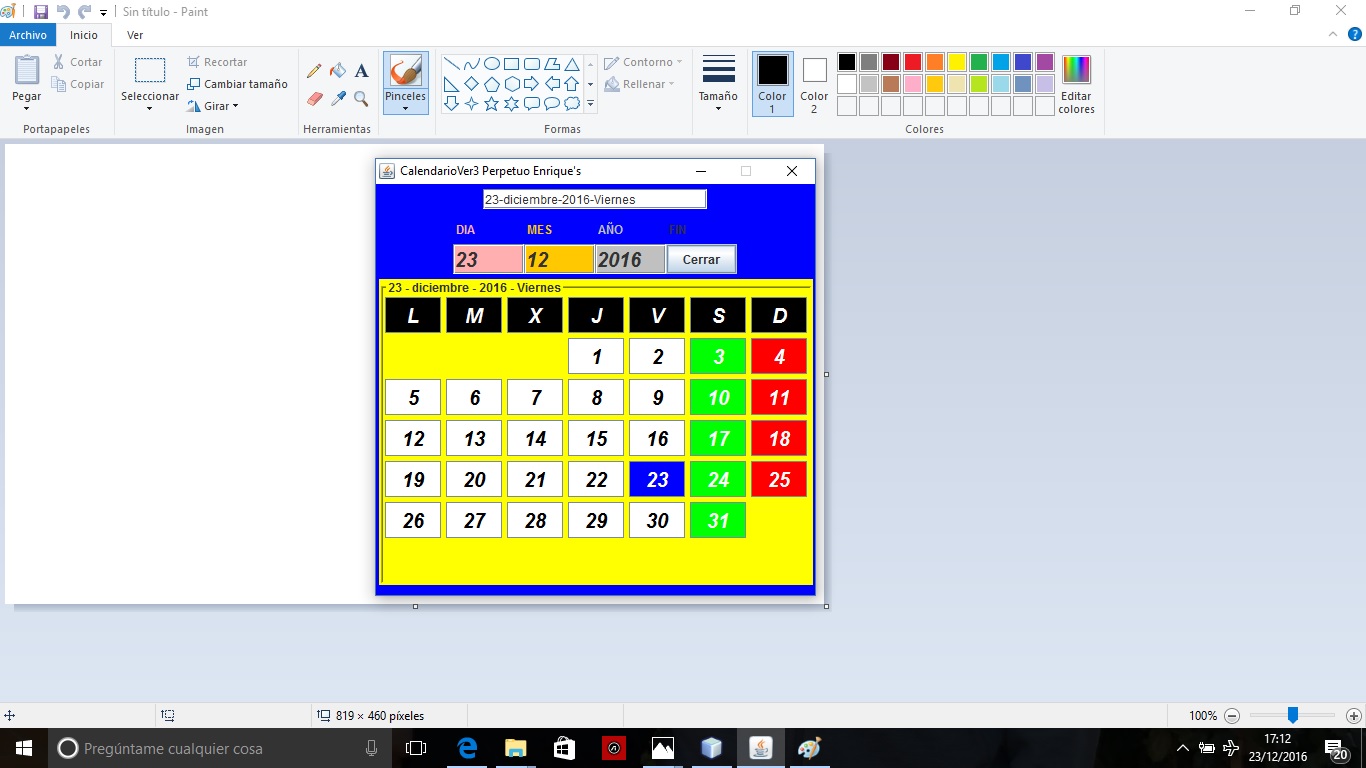
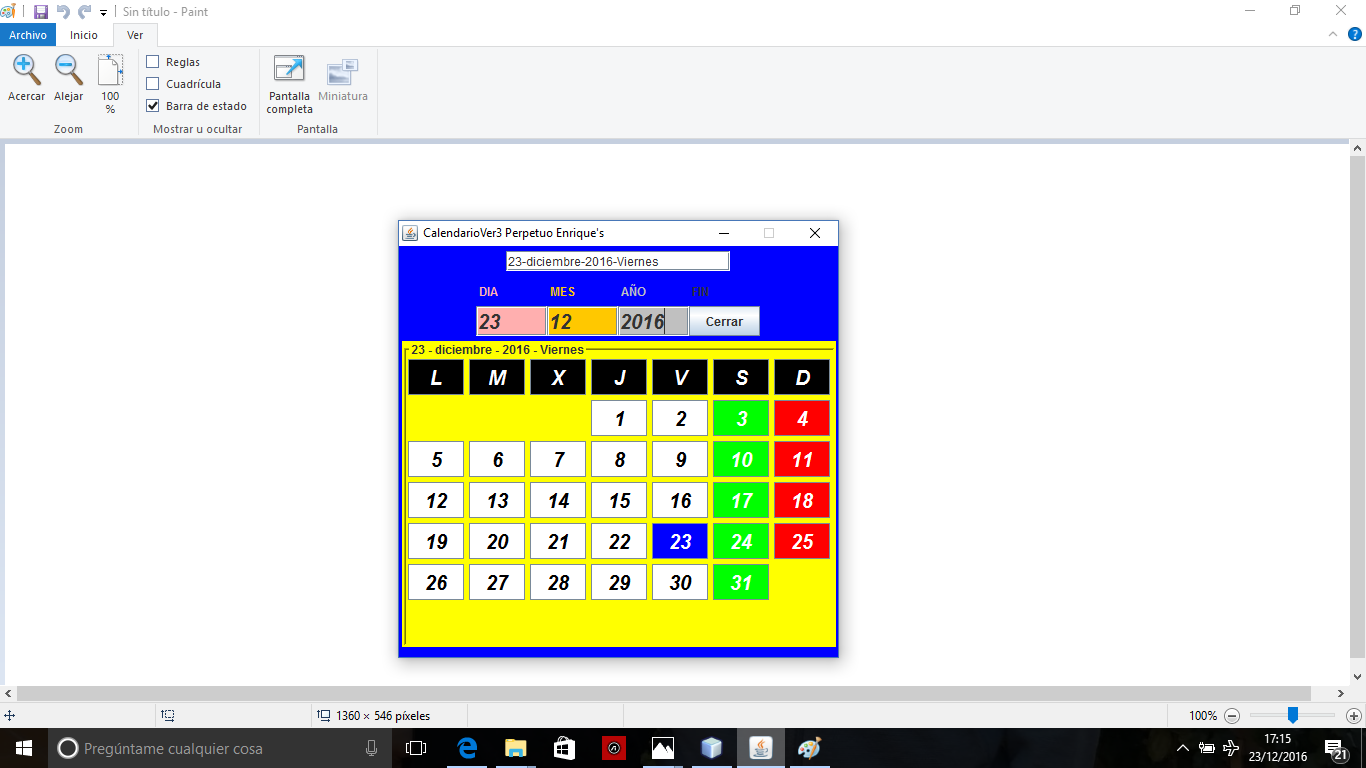
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.border.*;
public class CalendarioVer3 extends JFrame implements ActionListener, MouseWheelListener
{
Fecha f;
JPanel p,p1,p2;
JTextField tfFecha;
//JTextArea new taMes;
TitledBorder tBorder1;
JButton b1,b2,tbB[], tbD[] ;
//JButton aceptar;
JTextField tfd,tfm,tfa,tfh;
JLabel ldia,lmes, laño,l;
public CalendarioVer3()
{
Font font = new Font("Dialog",Font.BOLD|Font.ITALIC,20);
String[] mm= {"mes","Enero","Febrero","Marzo","Abril","Mayo","Junio","Julio","Agosto","Septiembre","Octubre","Noviembre","Diciembre"};
String[] dd= {"L","M","X","J","V","S","D"};
tBorder1 = new TitledBorder(BorderFactory.createBevelBorder(BevelBorder.LOWERED,Color.WHITE,Color.white,new Color(93, 93, 193),new Color(234)),"MES");
//tBorder1 = new TitledBorder(BorderFactory.createBevelBorder(BevelBorder.LOWERED,Color.white,Color.white,new Color(93, 93, 193),new Color(134, 134, 134)),"MES");
p= new JPanel();
p1 = new JPanel(new GridLayout(2,4));
p2 = new JPanel(new GridLayout(7,7,5,5));
p2.setBackground(Color.LIGHT_GRAY);//BLACK);//YELLOW);
p.setBackground(Color.BLUE);
tfFecha = new JTextField("Mueve rueda raton sobre mes-dia-año",20);
p2.setBorder(tBorder1);
p2.setMinimumSize(new Dimension(200,300));
p2.setBackground(Color.YELLOW);
//b1 = new JButton("LEER FECHA");
//b1.addActionListener(this);
b2 = new JButton("Cerrar");
b2.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e)
{ System.exit(-1);
}});
//aceptar= new JButton("ACEPTAR FECHA");
//aceptar.addActionListener(this);
tbD = new JButton[dd.length];
for(int i=0; i<tbD.length; i++)
{
tbD[i]=new JButton(dd[i]);
tbD[i].setForeground(Color.WHITE);
tbD[i].setBackground(Color.BLACK);
tbD[i].setVisible(false);
tbD[i].setFont(font);
p2.add(tbD[i]);
}
tbB = new JButton[42];
for(int i=0; i<tbB.length; i++)
{
tbB[i]=new JButton(" ");
tbB[i].setVisible(false);
tbB[i].addActionListener(this);
tbB[i].setFont(font);
p2.add(tbB[i]);
}
ldia= new JLabel(" DIA");
lmes= new JLabel(" MES");
laño= new JLabel(" AÑO");
l = new JLabel(" FIN ");
tfd= new JTextField("1",2);
tfm= new JTextField("1",2);
tfa= new JTextField("2009",3);
//tfd.setEditable(false);
//tfm.setEditable(false);
p1.setBackground(Color.BLUE);
tfd.setBackground(Color.PINK);
ldia.setForeground(Color.PINK);
tfm.setBackground(Color.ORANGE);
lmes.setForeground(Color.ORANGE);
tfa.setBackground(Color.LIGHT_GRAY);
laño.setForeground(Color.LIGHT_GRAY);
tfd.setFont(font);
tfm.setFont(font);
tfa.setFont(font);
/*
tfd.setToolTipText("para cambiar dia: mover rueda raton");
tfm.setToolTipText("mover rueda raton");
tfa.setToolTipText("mover rueda raton");
*/
tfd.addMouseWheelListener(this);
tfm.addMouseWheelListener(this);
tfa.addMouseWheelListener(this);
tfd.addActionListener(this);
tfm.addActionListener(this);
tfa.addActionListener(this);
//p.add(aceptar);
p.add(tfFecha);
p1.add(ldia);
p1.add(lmes);
p1.add(laño);
p1.add(l);
p1.add(tfd);
p1.add(tfm);
p1.add(tfa);
//p1.add(b1);
p1.add(b2);
p.add(p1);
p.add(p2);
getContentPane().add(p);
f = new Fecha();
}
private void escribirMes()
{
Fecha ff;
int i;
ff= new Fecha(1, f.mes, f.ano);// primer dia del mes
f.numSemComMes= f.getNumDiaSemana(ff); // cargar el dia de comienzo del mes
f.nombreMes=f.getNombreMes();
tBorder1 = new TitledBorder(BorderFactory.createBevelBorder(BevelBorder.LOWERED),f.dia+" - "+f.nombreMes+" - "+f.ano+" - "+f.getDiaSemana(f));
//tBorder1 = new TitledBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED/*LOWERED*/,Color.white,Color.white,new Color(93, 93, 193),new Color(134, 134, 134)),f.dia+" - "+f.nombreMes+" - "+f.ano+" - "+f.getDiaSemana(f));
p2.setBorder(tBorder1);
// p.setBackground(Color.BLACK);
for(i=0; i<tbD.length; i++)
tbD[i].setVisible(true);
//p2.setBackground(new Color((int)(Math.random()*10000)+ 1500));
for(i=0; i < f.numSemComMes; i++)
//tbB[i].setText("");
tbB[i].setVisible(false);
for(i=1; i <= f.diasMes(f.mes,f.ano); i++)
{
tbB[i+f.numSemComMes-1].setVisible(true);
tbB[i+f.numSemComMes-1].setText(String.valueOf(i));
// colores por defecto
tbB[i+f.numSemComMes-1].setBackground(Color.WHITE);//GRAY);
tbB[i+f.numSemComMes-1].setForeground(Color.BLACK);
if((i+f.numSemComMes)%7==0)
{
tbB[i+f.numSemComMes-1].setBackground(Color.RED);
tbB[i+f.numSemComMes-1].setForeground(Color.WHITE);
}
if((i+f.numSemComMes)%7==6)
{
tbB[i+f.numSemComMes-1].setBackground(Color.GREEN);//GRAY);
tbB[i+f.numSemComMes-1].setForeground(Color.WHITE);
}
if(i==f.dia)
{
tbB[i+f.numSemComMes-1].setBackground(Color.BLUE);
tbB[i+f.numSemComMes-1].setForeground(Color.WHITE);
}
}
for( ; i < tbB.length-f.numSemComMes+1; i++)
{
tbB[i+f.numSemComMes-1].setVisible(false);
}
tfFecha.setText(f.toString());
}
public void actionPerformed(ActionEvent ae)
{
JButton b;
if(ae.getSource() instanceof JButton)
{
// pulsando un dia, se pone cmo fecha actual.
b = (JButton) ae.getSource();
f.dia= Integer.parseInt(b.getLabel());
tfd.setText(b.getLabel());
escribirMes();
/* if(b==b1)
{
f.leerFecha();
tfd.setText(String.valueOf(f.dia));
tfm.setText(String.valueOf(f.mes));
tfa.setText(String.valueOf(f.ano));
tfFecha.setText(f.toString());
escribirMes();
}
if(b==b2)
System.exit(0);
if(b == aceptar)
{
actualizarFecha();
if(!f.fechaNoValida())
escribirMes();
}
*/
}
if(ae.getSource() instanceof JTextField)
{
f.dia=Integer.parseInt(tfd.getText());
f.mes=Integer.parseInt(tfm.getText());
f.ano=Integer.parseInt(tfa.getText());
escribirMes();
}
}
public void mouseWheelMoved(MouseWheelEvent e)
{
if(e.getSource() == tfa)
if (e.getWheelRotation()<=0)
tfa.setText(String.valueOf((Integer.parseInt(tfa.getText())+e.getScrollAmount()/3 )));
else
tfa.setText(String.valueOf((Integer.parseInt(tfa.getText())-e.getScrollAmount()/3 )));
if(e.getSource() == tfm)
if (e.getWheelRotation()<=0)
tfm.setText(String.valueOf(circular((Integer.parseInt(tfm.getText())+e.getScrollAmount()/3 ),12)));
else
tfm.setText(String.valueOf(circular((Integer.parseInt(tfm.getText())-e.getScrollAmount()/3 ),12)));
if(e.getSource() == tfd)
if (e.getWheelRotation()<=0)
tfd.setText(String.valueOf(circular((Integer.parseInt(tfd.getText())+e.getScrollAmount()/3 ),f.diasMes(f.mes,f.ano))));
else
tfd.setText(String.valueOf(circular((Integer.parseInt(tfd.getText())-e.getScrollAmount()/3 ),f.diasMes(f.mes,f.ano))));
actualizarFecha();
escribirMes();
}
public void actualizarFecha()
{
f.dia= Integer.parseInt(tfd.getText());
f.mes= Integer.parseInt(tfm.getText());
f.ano= Integer.parseInt(tfa.getText());
f.nombreMes = f.getNombreMes();
}
private int circular(int x,int n)
{
if(x>n)
return 1;
else
if(x<1)
return n;
else
return x;
}
public static void main(String [] ar)
{
CalendarioVer3 cal = new CalendarioVer3();
cal.setSize(445,440);
cal.setTitle("CalendarioVer3 Perpetuo Enrique's");
cal.setVisible(true);
cal.setResizable(false);
cal.addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent we){
System.exit(-1);
}
});
}
}
class Fecha
{
int dia,mes , ano;
private String diaSemana;
int numSemComMes;
int numDiaSem;
String nombreMes;
public Fecha()
{
dia=1;
mes=1;
ano =0;
}
public Fecha(int d, int m, int a)
{
dia = d;
mes = m;
ano = a;
//diaSemana = getDiaSemana(this);
//numDiaSem = getNumDiaSemana(this);
nombreMes = this.getNombreMes();
numSemComMes = -1;
}
/* public void leerFecha()
{
Integer oEnt;
do
{
try
{
dia = Integer.parseInt(JOptionPane.showInputDialog(null,"Introduce dia","LEER FECHA",JOptionPane.INFORMATION_MESSAGE));
mes = Integer.parseInt(JOptionPane.showInputDialog(null,"Introduce mes","LEER FECHA",JOptionPane.INFORMATION_MESSAGE));
ano = Integer.parseInt(JOptionPane.showInputDialog(null,"Introduce año","LEER FECHA",JOptionPane.INFORMATION_MESSAGE));
}
catch(NumberFormatException nfe){dia=1; mes=1;ano=1;}
nombreMes = getNombreMes();
diaSemana = getDiaSemana(this);
numDiaSem= getNumDiaSemana(this);
//System.out.println(" en LeerFecha" + numDiaSem);
} while(fechaNoValida());
}
*/
public boolean fechaNoValida()
{
boolean novale = true;
if(mes >=1 && mes <=12)
if(dia>=1 && dia <= diasMes(mes,ano))
novale=false;
return novale;
}
public int diasMes(int mes, int ano)
{
switch(mes)
{
case 1: case 3: case 5: case 7: case 8: case 10: case 12: return 31;
case 4: case 6: case 9: case 11: return 30;
case 2: if (bisiesto(ano)) return 29;
else return 28;
}
return -1;
}
private boolean bisiesto(int ano)
{
if((ano%4 == 0 && ano % 100 !=0) ||(ano%400==0))
return true;
else return false;
}
public String getNombreMes()
{
switch(mes)
{
case 1: nombreMes = "enero"; break;
case 2: nombreMes = "febrero"; break;
case 3: nombreMes = "marzo"; break;
case 4: nombreMes = "abril"; break;
case 5: nombreMes = "mayo"; break;
case 6:nombreMes = "junio"; break;
case 7: nombreMes = "julio"; break;
case 8: nombreMes = "agosto"; break;
case 9: nombreMes = "septiembre"; break;
case 10: nombreMes = "octubre"; break;
case 11: nombreMes = "noviembre"; break;
case 12: nombreMes = "diciembre"; break;
default: nombreMes="ERROR";
}
return nombreMes;
}
public String getDiaSemana(Fecha f1mes)
{
long f1,fref;
int x;
String ds;
Fecha fRef= new Fecha(2,3,2009); // lunes
x=contardias(fRef,f1mes); //
x = x % 7;
if(x<0) x = (x +7)%7;
switch(x)
{
case 0: ds = "Lunes"; break;
case 1: ds = "Martes"; break;
case 2: ds = "Miercoles"; break;
case 3: ds = "Jueves"; break;
case 4: ds = "Viernes"; break;
case 5: ds = "Sabado"; break;
case 6: ds = "Domingo"; break;
default: ds= "ERROR";
}
f1mes.diaSemana=ds;
return ds;
}
public int getNumDiaSemana(Fecha f1mes)
{
long f1,fref;
int x;
String ds;
Fecha fRef= new Fecha(2,3,2009); // lunes
x=contardias(fRef,f1mes); //
x = x % 7;
if(x<0) x = (x +7)%7;
f1mes.numDiaSem=x;
return x;
}
public int contardias(Fecha f1, Fecha f2)
{
int fi,ff, tot;
int a,m,mc,mf,ac,af;
Fecha f;
boolean neg;
neg = false;
fi = f1.dia + f1.mes * 100 + f1.ano * 10000;
ff = f2.dia + f2.mes * 100 + f2.ano * 10000;
if(fi > ff)
{
neg = true;
f = f1;
f1= f2;
f2= f;
}
tot=f2.dia-f1.dia;
for(a= f1.ano; a <= f2.ano; a++ )
{
if(a==f1.ano) mc = f1.mes;
else mc = 1;
if(a == f2.ano) mf = f2.mes-1;
else mf = 12;
for(m = mc ; m <= mf ; m++)
tot += diasMes(m,a);
}
if(neg) tot = -tot;
return tot;
}
public String toString()
{
return String.valueOf(dia)+"-"+nombreMes+"-"+String.valueOf(ano)+"-"+diaSemana;
}
}
Comentarios sobre la versión: Versión 3.0 (0)
No hay comentarios