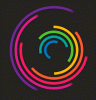
Ayuda con la creación de un archivo PDF
Publicado por Jonathan (1 intervención) el 05/05/2023 02:48:40
necesito crear un archivo que muestre la deuda que tiene un alumno en una institución educativa, solo que al llamar mi clase me señala un error que aun no he detectado...
si alguien me pudiera ayudar por favor.
les adjunto mi codigo...
si alguien me pudiera ayudar por favor.
les adjunto mi codigo...
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
package PDF;
import com.itextpdf.text.Chunk;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
//import conexion.conexionMysql;
import java.io.File;
import java.io.FileOutputStream;
//import java.sql.Connection;
import javax.swing.event.DocumentListener;
import javax.swing.event.UndoableEditListener;
import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import javax.swing.text.Element;
import javax.swing.text.Position;
import javax.swing.text.Segment;
public class Plantilla {
String nombre;
String apellido_p;
String apellido_m;
String licenciatura;
String fechaEmision;
String fechaLimite;
// float promedio;
// int matricula;
// int beca;
Document documento;
FileOutputStream archivo;
Paragraph titulo;
public Plantilla(String nombre, String apellido_p, String apellido_m, String licenciatura, String fechaEmision, String fechaLimite){
this.nombre = nombre;
this.apellido_p = apellido_p;
this.apellido_m = apellido_m;
this.licenciatura = licenciatura;
this.fechaEmision = fechaEmision;
this.fechaLimite = fechaLimite;
// this.promedio = promedio;
// this.matricula = matricula;
// this.beca = beca;
documento = new Document() {
@Override
public int getLength() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void addDocumentListener(DocumentListener listener) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void removeDocumentListener(DocumentListener listener) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void addUndoableEditListener(UndoableEditListener listener) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void removeUndoableEditListener(UndoableEditListener listener) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public Object getProperty(Object key) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void putProperty(Object key, Object value) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void remove(int offs, int len) throws BadLocationException {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void insertString(int offset, String str, AttributeSet a) throws BadLocationException {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public String getText(int offset, int length) throws BadLocationException {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void getText(int offset, int length, Segment txt) throws BadLocationException {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public Position getStartPosition() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public Position getEndPosition() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public Position createPosition(int offs) throws BadLocationException {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public Element[] getRootElements() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public Element getDefaultRootElement() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
@Override
public void render(Runnable r) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
};
titulo = new Paragraph("Papeleta");
}
public void crearPlantilla(){
try {
archivo = new FileOutputStream(nombre + ".pdf");
PdfWriter.getInstance((com.itextpdf.text.Document) documento, archivo);
((StreamConnection)documento).open();
//// documento.open();
titulo.setAlignment(1);
((StreamConnection)documento).add(titulo);
((StreamConnection)documento).add(new Paragraph("Nombre del Alumno: \n" + nombre));
((StreamConnection)documento).add(Chunk.NEWLINE);
((StreamConnection)documento).add(new Paragraph("Apellidos: " + apellido_p + apellido_m));
((StreamConnection)documento).add(Chunk.NEWLINE);
((StreamConnection)documento).add(new Paragraph("Licenciatura/Maestria: " + licenciatura));
Paragraph subTitulo = new Paragraph("Datos de la Mensualidad");
subTitulo.setAlignment(3);
((StreamConnection)documento).add(new Paragraph(subTitulo));
((StreamConnection)documento).add(Chunk.NEWLINE);
((StreamConnection)documento).add(new Paragraph("Fecha de Emisión" + fechaEmision));
((StreamConnection)documento).add(Chunk.NEWLINE);
((StreamConnection)documento).add(new Paragraph("Fecha Limite de Pago" + fechaLimite));
((StreamConnection)documento).close();
// documento.close();
// FileOutputStream outputStream = new FileOutputStream(new File());
// outputStream.close();
// workbook.close();
} catch (Exception e) {
System.out.println("error al crear el archivo " + e);
}
}
private static class StreamConnection {
public StreamConnection() {
}
private void open() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
private void add(Paragraph titulo) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
private void add(Chunk NEWLINE) {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
private void close() {
throw new UnsupportedOperationException("Not supported yet."); // Generated from nbfs://nbhost/SystemFileSystem/Templates/Classes/Code/GeneratedMethodBody
}
}
}
Valora esta pregunta


0