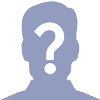
por favor me podrian ayudar en un ejercicio de java en programacion Orientada a Objetos
Publicado por hernan (6 intervenciones) el 25/02/2023 12:25:29
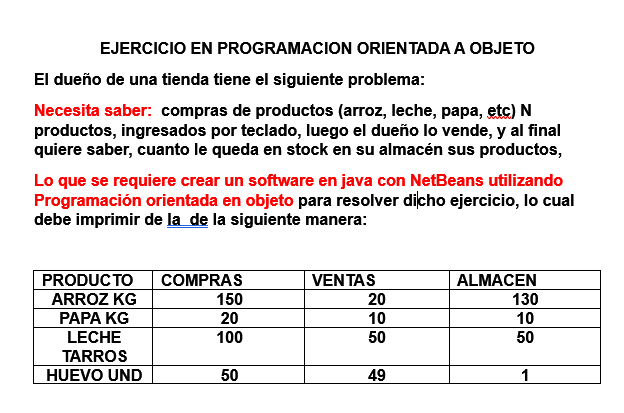
Valora esta pregunta


0
public class Producto {
private String nombre;
private String formato; //Kg, tarros, botellas, unidades....
private int compras;
private int ventas;
public Producto(String nombre, String formato) {
this.nombre = nombre;
this.formato = formato;
compras = 0;
ventas = 0;
}
public String getNombre() {
return nombre;
}
public String getFormato() {
return formato;
}
public String getDescripcion() {
return nombre + " " + formato;
}
public int getCompras() {
return compras;
}
public int getVentas() {
return ventas;
}
public int getStock() {
return compras - ventas;
}
public void comprar(int cantidad) {
compras += cantidad;
}
public boolean vender(int cantidad) {
if (cantidad > getStock())
return false; //No hay suficiente stock para cubrir las unidades de venta
else {
ventas += cantidad;
return true;
}
}
}
import java.util.ArrayList;
import java.util.Scanner;
public class Tienda {
private static ArrayList<Producto> productos = new ArrayList<Producto>();
private static Scanner teclado = new Scanner(System.in);
public static void main(String[] args) {
int opcion = 0;
while (opcion != 4) {
System.out.println("\n\t\tMENU TIENDA");
System.out.println("\t\t---- ------\n");
System.out.println("(1) -- Comprar Producto");
System.out.println("(2) -- Vender Producto");
System.out.println("(3) -- Listar stock de Productos");
System.out.println("(4) -- TERMINAR PROGRAMA");
System.out.print("Opcion: ");
opcion = Integer.parseInt(teclado.nextLine());
switch(opcion) {
case 1:
comprarProducto();
break;
case 2:
venderProducto();
break;
case 3:
listarStock();
break;
case 4:
System.out.println("\n\t\tFIN DE PROGRAMA");
break;
default:
System.out.println("Opción equivocada");
}
}
}
private static void comprarProducto() {
System.out.println("\n\t\tCOMPRAR PRODUCTO");
System.out.print("Nombre de producto: ");
String nombre = teclado.nextLine().toUpperCase();
System.out.print("Formato de producto(kg,unid,tarros,...): ");
String formato = teclado.nextLine();
System.out.print("Cantidad: ");
int cantidad = Integer.parseInt(teclado.nextLine());
//Comprobamos si ya existe producto, o es uno nuevo que hay que registrar
Producto buscar = null;
for (Producto prd: productos) {
if (prd.getNombre().equals(nombre)) {
buscar = prd;
break; //Búsqueda finalizada
}
}
if (buscar == null) { //Es nuevo, hay que registrarlo
Producto nuevo = new Producto(nombre, formato);
nuevo.comprar(cantidad);
productos.add(nuevo);
System.out.println("\n\tNuevo producto registrado\n");
}
else { //Ya existe en el registro de productos
buscar.comprar(cantidad);
System.out.println("\n\tCompra registrada\n");
}
}
private static void venderProducto() {
System.out.println("\n\t\tVENDER PRODUCTO");
System.out.print("Nombre de producto: ");
String nombre = teclado.nextLine().toUpperCase();
//Buscamos el producto
Producto buscar = null;
for (Producto prd: productos) {
if (prd.getNombre().equals(nombre)) {
buscar = prd;
break; //Búsqueda finalizada
}
}
if (buscar == null) //No existe, programa volverá al menu principal sin hacer nada
System.out.println("No se encuentra producto con el nombre: " + nombre);
else {//Existe, pedimos cantidad
System.out.print("Indique cantidad de " + buscar.getFormato() + " a vender: ");
int cantidad = Integer.parseInt(teclado.nextLine());
if (buscar.vender(cantidad))
System.out.println("\n\tVenta registrada\n");
else
System.out.println("\n\tNo hay stock para cubrir la venta\n");
}
}
private static void listarStock() {
System.out.println("\n\t\tSTOCK PRODUCTOS");
System.out.println("\t\t----- ---------\n");
if (productos.isEmpty())
System.out.println("\n\tNo hay productos registrados\n");
else {
System.out.printf("%20s\t%7s\t%7s\t%7s\n", "PRODUCTO ", "COMPRAS", "VENTAS", "ALMACEN");
for (Producto prd: productos)
System.out.printf("%20s\t%7d\t%7d\t%7d\n", prd.getDescripcion(), prd.getCompras(),
prd.getVentas(), prd.getStock());
}
}
}